Problems
C++ Types
- What is a C++ type?
- List as many C++ types as you can.
- Are pointers and references a C++ type?
Pointers
- What does a pointer hold?
- Write a line of code that declares a pointer to a double.
References
- What is a reference?
- Write a line of code that initializes a reference to a float variable named
x
.
Referencing and dereferencing
- What does the reference operator do?
- What does the dereference operator do?
- Write a line of code that initializes a pointer to the address of an integer variable named
y
- The following program performs pointer arithmetic. In general terms, what should the following program output?
1
2
3
4
5
6
7
8
9
10
11
12
13 | #include <iostream>
using namespace std;
int main() {
int var = 5;
int* ptr = &var;
cout << var << endl;
cout << &var << endl;
cout << ptr << endl;
cout << *ptr << endl;
cout << &ptr << endl;
}
|
- The following program performs reference arithmetic. In general terms, what should the following program output?
1
2
3
4
5
6
7
8
9
10
11
12 | #include <iostream>
using namespace std;
int main() {
int var = 5;
int& ref = var;
cout << var << endl;
cout << &var << endl;
cout << ref << endl;
cout << &ref << endl;
}
|
Stack
- What is the stack used for?
- What are the limitations of the stack?
- Fill out the following table with respect to the program from question
4 in Referencing and Dereferencing
and question 5 in Referencinng and Dereferencing
Question 4
Variable name(s) | Value | Address |
| | |
Question 5
Variable name(s) | Value | Address |
| | |
Heap
- What is heap used for?
- What benefits does the heap have?
- What are the downsides to using the heap?
- What does it mean to dynamically allocate?
- What data type does the
new
keyword return? - Why do pointers need to be used with dynamic memory?
- Write a line of code to deallocate the memory referenced by the pointer
ptr
. - Fill out the stack and heap tables below with respect to following program.
| #include <iostream>
using namespace std;
int main() {
int *iptr = nullptr;
iptr = new int(5);
double *dptr;
double dvar = 5.8;
dptr = &dvar;
}
|
Stack
Variable name(s) | Value | Address |
| | |
| | |
| | |
Heap
Passing by Value vs Passing by Reference
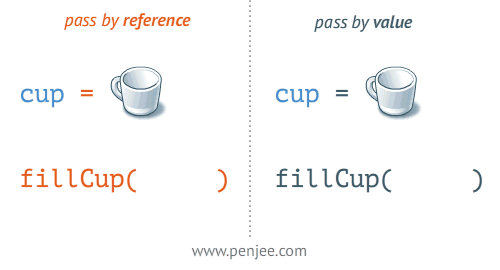
- What does it mean to pass by reference?
- What does it mean to pass by value?
- What are the advantages of passing by reference?
- What are the advantages of passing by value?
- Consider the following functions:
| void swap_a(int a, int b) {
int temp = a;
a = b;
b = temp;
}
void swap_b(int &a, int &b) {
int temp = a;
a = b;
b = temp;
}
|
What does the following program output? Does it pass by value or by reference? | #include <iostream>
using namespace std;
int main() {
int x(2), y(3);
cout << x << " " << y << endl;
swap_a(x, y);
cout << x << " " << y << endl;
}
|
What does the following program output? Does it pass by value or by reference?
| #include <iostream>
using namespace std;
int main() {
int x(2), y(3);
cout << x << " " << y << endl;
swap_b(x, y);
cout << x << " " << y << endl;
}
|
Classes and Objects
- What are the differences between classes and objects?
- What are constructors and destructors?
- What is public and private?
- Design a class with the following details:
- class for cars
- private attributes: brand, lisencse plate
- public methods: set brand, set license plate, get brand, get license plate
- Create two objects from the class above. The first having the brand Ford and license plate number 1234567. The second having the brand name BMW and license plate 7654321.